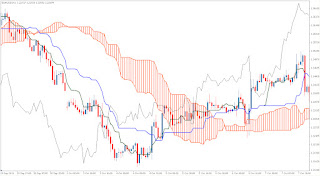 |
Ichimoku |
 |
Ichimoku |
 |
Ichimoku |
 |
Ichimoku |
 |
Ichimoku |
 |
Ichimoku |
 |
Ichimoku |
 |
Ichimoku |
Ichimoku Kinko Hyo is a technical indicator, which nicely illustrates the long-term trend and also warns the trader to buy and sell signals. Ichimoku Kinko Hyo shows four time frames - Tenkan-sen, Kijun-sen, Chinku Span, Senkou Span I and II.
Tenkan-sen, in our case green, shows average price for the first period. Tenkan-sen = sum of maximum and minimum of this period / 2.
Kijun-sen, in our case blue, indicates average price for the second period. Kijun-sen = sum of maximum and minimum of second period / 2.
Chinku Span, in our case black, displays trend, respectively displays the closing price of the current bar moved backwards in the size of the second period. If Chinkou Span crosses the price, it is the signal for purchase or sale. If the price in the cloud, it is not market trends. If the price is above the clouds - shopping trend, if the cloud - sales trend.
Senku Span I ( In our case pink) shows the middle of distance between the previous two lines moved forward in the length of the second time period and Senkou Span II ( In our case pink) shows average price of the third period moved forward in the size of the second time period.
Ichimoku Kinko Hyo MQ4 Code Base (Copy Code)
//+------------------------------------------------------------------+
//| Ichimoku Kinko Hyo.mq4 |
//| Copyright © 2004, MetaQuotes Software Corp. |
//| http://www.metaquotes.net/ |
//+------------------------------------------------------------------+
#property copyright "Copyright © 2004, MetaQuotes Software Corp."
#property link "http://www.metaquotes.net/"
#property indicator_chart_window
#property indicator_buffers 7
#property indicator_color1 Red
#property indicator_color2 Blue
#property indicator_color3 SandyBrown
#property indicator_color4 Thistle
#property indicator_color5 Lime
#property indicator_color6 SandyBrown
#property indicator_color7 Thistle
//---- input parameters
extern int Tenkan=9;
extern int Kijun=26;
extern int Senkou=52;
//---- buffers
double Tenkan_Buffer[];
double Kijun_Buffer[];
double SpanA_Buffer[];
double SpanB_Buffer[];
double Chinkou_Buffer[];
double SpanA2_Buffer[];
double SpanB2_Buffer[];
//----
int a_begin;
//+------------------------------------------------------------------+
//| Custom indicator initialization function |
//+------------------------------------------------------------------+
int init()
{
//----
SetIndexStyle(0,DRAW_LINE);
SetIndexBuffer(0,Tenkan_Buffer);
SetIndexDrawBegin(0,Tenkan-1);
SetIndexLabel(0,"Tenkan Sen");
//----
SetIndexStyle(1,DRAW_LINE);
SetIndexBuffer(1,Kijun_Buffer);
SetIndexDrawBegin(1,Kijun-1);
SetIndexLabel(1,"Kijun Sen");
//----
a_begin=Kijun; if(a_begin<Tenkan) a_begin=Tenkan;
SetIndexStyle(2,DRAW_HISTOGRAM,STYLE_DOT);
SetIndexBuffer(2,SpanA_Buffer);
SetIndexDrawBegin(2,Kijun+a_begin-1);
SetIndexShift(2,Kijun);
SetIndexLabel(2,NULL);
SetIndexStyle(5,DRAW_LINE,STYLE_DOT);
SetIndexBuffer(5,SpanA2_Buffer);
SetIndexDrawBegin(5,Kijun+a_begin-1);
SetIndexShift(5,Kijun);
SetIndexLabel(5,"Senkou Span A");
//----
SetIndexStyle(3,DRAW_HISTOGRAM,STYLE_DOT);
SetIndexBuffer(3,SpanB_Buffer);
SetIndexDrawBegin(3,Kijun+Senkou-1);
SetIndexShift(3,Kijun);
SetIndexLabel(3,NULL);
SetIndexStyle(6,DRAW_LINE,STYLE_DOT);
SetIndexBuffer(6,SpanB2_Buffer);
SetIndexDrawBegin(6,Kijun+Senkou-1);
SetIndexShift(6,Kijun);
SetIndexLabel(6,"Senkou Span B");
//----
SetIndexStyle(4,DRAW_LINE);
SetIndexBuffer(4,Chinkou_Buffer);
SetIndexShift(4,-Kijun);
SetIndexLabel(4,"Chinkou Span");
//----
return(0);
}
//+------------------------------------------------------------------+
//| Ichimoku Kinko Hyo |
//+------------------------------------------------------------------+
int start()
{
int i,k;
int counted_bars=IndicatorCounted();
double high,low,price;
//----
if(Bars<=Tenkan || Bars<=Kijun || Bars<=Senkou) return(0);
//---- initial zero
if(counted_bars<1)
{
for(i=1;i<=Tenkan;i++) Tenkan_Buffer[Bars-i]=0;
for(i=1;i<=Kijun;i++) Kijun_Buffer[Bars-i]=0;
for(i=1;i<=a_begin;i++) { SpanA_Buffer[Bars-i]=0; SpanA2_Buffer[Bars-i]=0; }
for(i=1;i<=Senkou;i++) { SpanB_Buffer[Bars-i]=0; SpanB2_Buffer[Bars-i]=0; }
}
//---- Tenkan Sen
i=Bars-Tenkan;
if(counted_bars>Tenkan) i=Bars-counted_bars-1;
while(i>=0)
{
high=High[i]; low=Low[i]; k=i-1+Tenkan;
while(k>=i)
{
price=High[k];
if(high<price) high=price;
price=Low[k];
if(low>price) low=price;
k--;
}
Tenkan_Buffer[i]=(high+low)/2;
i--;
}
//---- Kijun Sen
i=Bars-Kijun;
if(counted_bars>Kijun) i=Bars-counted_bars-1;
while(i>=0)
{
high=High[i]; low=Low[i]; k=i-1+Kijun;
while(k>=i)
{
price=High[k];
if(high<price) high=price;
price=Low[k];
if(low>price) low=price;
k--;
}
Kijun_Buffer[i]=(high+low)/2;
i--;
}
//---- Senkou Span A
i=Bars-a_begin+1;
if(counted_bars>a_begin-1) i=Bars-counted_bars-1;
while(i>=0)
{
price=(Kijun_Buffer[i]+Tenkan_Buffer[i])/2;
SpanA_Buffer[i]=price;
SpanA2_Buffer[i]=price;
i--;
}
//---- Senkou Span B
i=Bars-Senkou;
if(counted_bars>Senkou) i=Bars-counted_bars-1;
while(i>=0)
{
high=High[i]; low=Low[i]; k=i-1+Senkou;
while(k>=i)
{
price=High[k];
if(high<price) high=price;
price=Low[k];
if(low>price) low=price;
k--;
}
price=(high+low)/2;
SpanB_Buffer[i]=price;
SpanB2_Buffer[i]=price;
i--;
}
//---- Chinkou Span
i=Bars-1;
if(counted_bars>1) i=Bars-counted_bars-1;
while(i>=0) { Chinkou_Buffer[i]=Close[i]; i--; }
//----
return(0);
}
//+------------------------------------------------------------------+
Ichimoku Kinko Hyo MQ5 Code Base (Copy Code)
//+------------------------------------------------------------------+
//| Ichimoku.mq5 |
//| Copyright 2009, MetaQuotes Software Corp. |
//| http://www.mql5.com |
//+------------------------------------------------------------------+
#property copyright "2009, MetaQuotes Software Corp."
#property link "http://www.mql5.com"
#property description "Ichimoku Kinko Hyo"
//--- indicator settings
#property indicator_chart_window
#property indicator_buffers 5
#property indicator_plots 4
#property indicator_type1 DRAW_FILLING
#property indicator_type2 DRAW_LINE
#property indicator_type3 DRAW_LINE
#property indicator_type4 DRAW_LINE
#property indicator_color1 SandyBrown,Thistle
#property indicator_color2 Red
#property indicator_color3 Blue
#property indicator_color4 Lime
#property indicator_label1 "Senkou Span A;Senkou Span B"
#property indicator_label2 "Tenkan-sen"
#property indicator_label3 "Kijun-sen"
#property indicator_label4 "Chinkou Span"
//--- input parameters
input int InpTenkan=9; // Tenkan-sen
input int InpKijun=26; // Kijun-sen
input int InpSenkou=52; // Senkou Span B
//--- indicator buffers
double ExtTenkanBuffer[];
double ExtKijunBuffer[];
double ExtSpanABuffer[];
double ExtSpanBBuffer[];
double ExtChinkouBuffer[];
//+------------------------------------------------------------------+
//| Custom indicator initialization function |
//+------------------------------------------------------------------+
void OnInit()
{
//--- indicator buffers mapping
SetIndexBuffer(0,ExtSpanABuffer,INDICATOR_DATA);
SetIndexBuffer(1,ExtSpanBBuffer,INDICATOR_DATA);
SetIndexBuffer(2,ExtTenkanBuffer,INDICATOR_DATA);
SetIndexBuffer(3,ExtKijunBuffer,INDICATOR_DATA);
SetIndexBuffer(4,ExtChinkouBuffer,INDICATOR_DATA);
//---
IndicatorSetInteger(INDICATOR_DIGITS,_Digits+1);
//--- sets first bar from what index will be drawn
PlotIndexSetInteger(0,PLOT_DRAW_BEGIN,InpSenkou);
PlotIndexSetInteger(1,PLOT_DRAW_BEGIN,InpTenkan);
PlotIndexSetInteger(2,PLOT_DRAW_BEGIN,InpKijun);
//--- lines shifts when drawing
PlotIndexSetInteger(0,PLOT_SHIFT,InpKijun);
PlotIndexSetInteger(3,PLOT_SHIFT,-InpKijun);
//--- change labels for DataWindow
PlotIndexSetString(1,PLOT_LABEL,"Tenkan-sen("+string(InpTenkan)+")");
PlotIndexSetString(2,PLOT_LABEL,"Kijun-sen("+string(InpKijun)+")");
//--- sets drawing line empty value
PlotIndexSetDouble(0,PLOT_EMPTY_VALUE,0.0);
PlotIndexSetDouble(1,PLOT_EMPTY_VALUE,0.0);
PlotIndexSetDouble(2,PLOT_EMPTY_VALUE,0.0);
PlotIndexSetDouble(3,PLOT_EMPTY_VALUE,0.0);
//--- initialization done
}
//+------------------------------------------------------------------+
//| get highest value for range |
//+------------------------------------------------------------------+
double Highest(const double&array[],int range,int fromIndex)
{
double res=0;
//---
res=array[fromIndex];
for(int i=fromIndex;i>fromIndex-range && i>=0;i--)
{
if(res<array[i]) res=array[i];
}
//---
return(res);
}
//+------------------------------------------------------------------+
//| get lowest value for range |
//+------------------------------------------------------------------+
double Lowest(const double&array[],int range,int fromIndex)
{
double res=0;
//---
res=array[fromIndex];
for(int i=fromIndex;i>fromIndex-range && i>=0;i--)
{
if(res>array[i]) res=array[i];
}
//---
return(res);
}
//+------------------------------------------------------------------+
//| Ichimoku Kinko Hyo |
//+------------------------------------------------------------------+
int OnCalculate(const int rates_total,const int prev_calculated,
const datetime &Time[],
const double &Open[],
const double &High[],
const double &Low[],
const double &Close[],
const long &TickVolume[],
const long &Volume[],
const int &Spread[])
{
int limit;
//---
if(prev_calculated==0) limit=0;
else limit=prev_calculated-1;
//---
for(int i=limit;i<rates_total;i++)
{
ExtChinkouBuffer[i]=Close[i];
//--- tenkan sen
double high=Highest(High,InpTenkan,i);
double low=Lowest(Low,InpTenkan,i);
ExtTenkanBuffer[i]=(high+low)/2.0;
//--- kijun sen
high=Highest(High,InpKijun,i);
low=Lowest(Low,InpKijun,i);
ExtKijunBuffer[i]=(high+low)/2.0;
//--- senkou span a
ExtSpanABuffer[i]=(ExtTenkanBuffer[i]+ExtKijunBuffer[i])/2.0;
//--- senkou span b
high=Highest(High,InpSenkou,i);
low=Lowest(Low,InpSenkou,i);
ExtSpanBBuffer[i]=(high+low)/2.0;
}
//--- done
return(rates_total);
}
//+------------------------------------------------------------------+